Singletonパターン Java
デザインパターンの一つであるFactoryMethodパターンについて記述する。
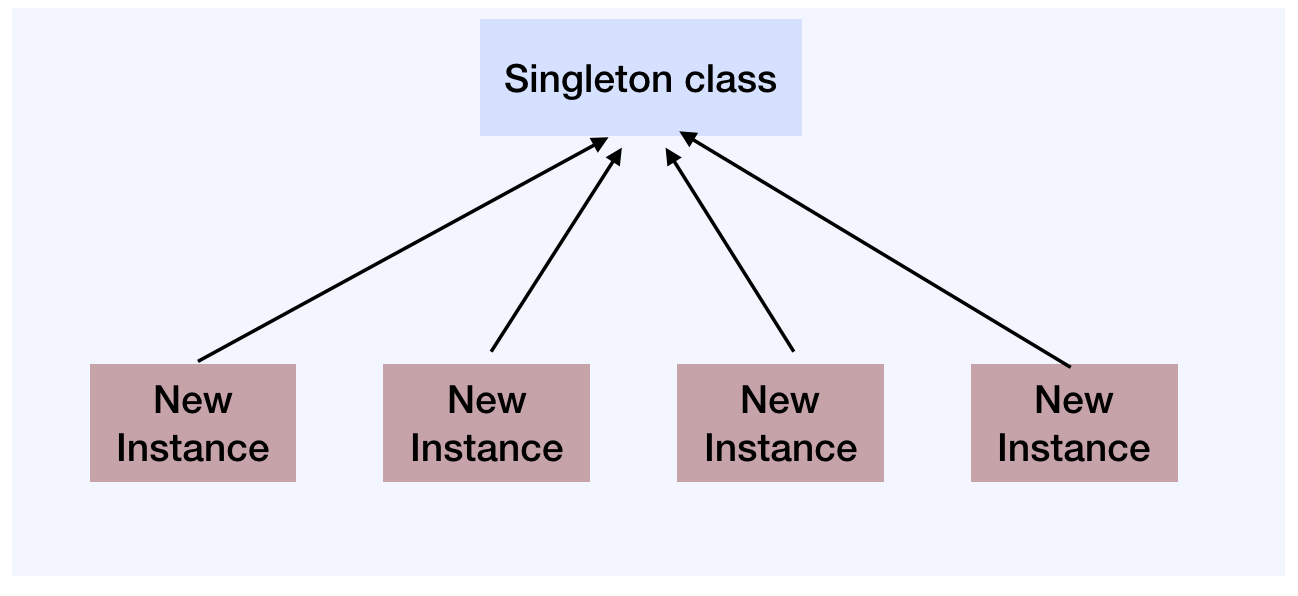
なぜSingletonパターン?
Singletonパターンは、常に一つのインスタンスしか存在しないアーキテクチャ。
アプリケーション設計でSingletonパターンを意識すれば、無駄に生成しているインスタンス等があれば省略することができる。
JavaのSpringのDIはSingletonになっている。
Singletonパターンの実装ルールをあげる。
- 自分自身のインスタンスは、クラスフィールド かつ privateで定義する
- 外部クラスからSingletonクラスを生成しない
- Singletonのクラスで、1の変数を返却するメソッドを実装する。インスタンスを使い回す
サンプルコード
Mainクラス。
1 2 3 4 5 6 7 8 9 10 11 12
| public class Main { public static void main(String[] args) { Singleton instance1 = Singleton.getInstance(); Singleton instance2 = Singleton.getInstance();
if (instance1 == instance2) { System.out.println("Same Instance."); } else { System.out.println("Not same Instance."); } } }
|
Singletonクラス。
パターン1。
1 2 3 4 5 6 7 8 9 10 11 12
| public class Singleton {
private static Singleton singleton = new Singleton(); private Singleton() { System.out.println("Create Instance"); } public static Singleton getInstance() { return singleton; } }
|
Singleton class.
パターン2。
1 2 3 4 5 6 7 8 9 10
| class Singleton { static Singleton instance = null; public static synchronized Singleton getInstance() { if (instance == null) { instance = new Singleton(); } return instance; } }
|